InitialDPSMS Lua Service
Introduction
The InitialDPSMSLuaService is a service for initiating Lua scripts running within the LogicApp.
The InitialDPSMSLuaService receives a InitialDPSMS
operation contained in a TCAP-RECV
message from an instance of the
SigtranApp which is
configured to receive TCAP messages from an external client.
During the transaction, the InitialDPSMSLuaService communicates with the SigtranApp using the
TCAP-SEND
and TCAP-RECV
messages. See the definition of the
TCAP-… messages.
Message Flows
The following message flows show the direct and reservation mechanisms.
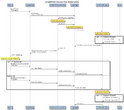
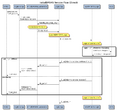
Configuring InitialDPSMSLuaService
The InitialDPSMSLuaService is configured within a LogicApp.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp" stderr_debug="0">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
<parameter name="trace_level" value="1"/>
<parameter name="default_lua_lib_path" value="../../n2scp/lua/lib/?.lua;../lua/lib/?.lua"/>
</parameters>
<config>
<services>
<service module="SigtranApp::InitialDPSMSLuaService" libs="../../n2scp/apps/sigtran/lib" script_dir="../../n2scp/test/regression/set7-SMS/logic_app">
<triggers>
<trigger script_key="camel_sms"/>
</triggers>
</service>
</services>
<normalisation>
<sender>
<rule leading="00" trim="2" prepend=""/>
<rule leading="0" trim="1" prepend="64"/>
</sender>
<destination>
<rule leading="00" trim="2" prepend=""/>
<rule leading="0" trim="1" prepend="64"/>
</destination>
</normalisation>
<denormalisation>
<sender>
<rule nature="international" min_len="6"/>
<rule nature="unknown"/>
</sender>
<destination>
<rule nature="international" min_len="6"/>
<rule nature="unknown"/>
</destination>
</denormalisation>
</config>
</application>
...
</application>
...
</n2svcd>
In addition to the Common LogicApp Service Configuration, note the following specific attribute notes, and service-specific attributes.
Under normal installation, the following service
attributes apply:
Parameter Name | Type | XML Type | Description |
---|---|---|---|
module
|
String | Attribute |
[Required] The module name containing the Lua Service code: SigtranApp::InitialDPSMSLuaService
|
libs
|
String | Element |
Location of the module for InitialDPSMSLuaService.(Default: ../../n2scp/apps/sigtran/lib )
|
script_dir
|
String | Attribute | [Required] The directory containing scripts used by this service. |
default_release_cause
|
Integer | Element |
The release cause to use in the ReleaseSMS sent by us, if not explicitly specified by service logic.(Default = 1 ).
|
default_submit_timeout
|
Float | Element |
The default duration (in seconds) after which the script will resume if no EventReportSMS operation is received when using the termination_attempt method.This timeout applies only for InitialDPSMS processing with eventTypeSMS = 1 (SMS Collected Info).(Default = 10 seconds).
|
default_deliver_timeout
|
Float | Element |
The default duration (in seconds) after which the script will resume if no EventReportSMS operation is received when using the termination_attempt method.This timeout applies only for InitialDPSMS processing with eventTypeSMS = 11 (SMS Delivery Requested).(Default = 10 seconds).
|
timeout_policy
|
disarm_end / empty_end / abort
|
Element |
The behavior to perform if the SMSC fails to respond within the expected timeout when we send
RequestReportSMSEvent with ConnectSMS /ContinueSMS .disarm_end - We send RequestReportSMSEvent with EDPs (Monitor Mode Transparent) in a TCAP END message.empty_end - We send an empty TCAP END message.abort - We send a TCAP ABORT message.(Default = disarm_end ).
|
.triggers
|
Array | Element |
Array of trigger elements specifying Lua scripts to run for InitialDPSMS Inbound Transactions.
|
.trigger
|
Object | Element | Provisions a Lua script to run for a InitialDPSMS Inbound Transaction for received InitialDPSMS operations. |
Script Trigger Rules
Each InitialDPSMS trigger rule defines the name of a script which is ready to handle an inbound InitialDPSMS Transaction.
Note that destination and calling addresses may contain hex digits A-F, and the
matching is for destination_prefix
and calling_prefix
is case-insensitive.
Each trigger
Object in the config
.triggers
Array is configured as follows.
Parameter Name | Type | XML Type | Description |
---|---|---|---|
ssn
|
1 -255
|
Attribute |
This trigger applies for only InitialDPSMS to this exact SCCP subsystem number. (Default = Trigger applies to all SCCP subsystem numbers). |
service_key
|
Integer | Attribute |
This trigger applies for only InitialDPSMS to this exact service key. (Default = Trigger applies to all InitialDPSMS). |
destination
|
Hex Digits | Attribute |
This trigger applies for only InitialDPSMS to this exact destination subscriber number digits (in InitialDPSMS). (Default = Trigger applies to all InitialDPSMS). |
destination_prefix
|
Hex Digits | Attribute |
This trigger applies for only InitialDPSMS to destination subscriber number digits (in InitialDPSMS) with this prefix. (Default = Trigger applies to all InitialDPSMS). |
calling
|
Hex Digits | Attribute |
This trigger applies for only InitialDPSMS from this exact calling party number digits (in InitialDPSMS). (Default = Trigger applies to all InitialDPSMS). |
calling_prefix
|
Integer | Attribute |
This trigger applies for only InitialDPSMS from calling party number digits (in InitialDPSMS) with this prefix. (Default = Trigger applies to all InitialDPSMS). |
script_key
|
String | Attribute |
The name for the Lua script to load (excluding the ".lua" or ".lc" suffix). The script should reside in the configured script_dir directory.
|
Script Selection (InitialDPSMS Transaction Request)
The Lua script selection to execute for a InitialDPSMS takes into consideration the received content of the inbound TCAP_BEGIN transaction and/or the SCCP addressing information.
The destination address for matching is:
- The InitialDPSMS Destination Subscriber Number Digits.
The calling address for matching is:
- The InitialDPSMS Calling Party Number Digits.
The InitialDPSMS Lua Service will iterate the configured trigger
entries in the sequence
in which they are configured and find the first trigger which matches the parameters of the
received message.
Refer to the LogicApp configuration for more information on directories, library paths, and script caching parameters.
Script Global Variables
Scripts run with this service have access to the Common Lua Service Global Variables.
There are no service-specific global variables.
Number Normalisation
Scripts run with this service may use N2SVCD number normalisation, e.g.:
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp" stderr_debug="0">
...
<config>
<services>
<service module="SigtranApp::InitialDPSMSLuaService" ... />
</services>
<normalisation>
<common>
<rule .../>
</common>
<sender>
<rule .../>
</sender>
<destination>
<rule ..."/>
</destination>
</normalisation>
<denormalisation>
<common>
<rule .../>
</common>
<sender>
<rule .../>
</sender>
<destination>
<rule .../>
</destination>
</denormalisation>
</config>
</application>
...
</application>
...
</n2svcd>
Rules in the sender
block are treated as
MAP address strings and apply to:
- Normalisation:
- the
callingPartyNumber
in theInitialDPSMS
message.
- the
- Deormalisation:
- The
callingPartysNumber
in theConnectSMS
message. - The
sMSCAddress
in theConnectSMS
message.
- The
Rules in the destination
block are treated as
GSM called party BCD numbers and apply to:
- Normalisation:
- the
destinationSubscriberNumber
in theInitialDPSMS
message.
- the
- Deormalisation:
- The
destinationSubscriberNumber
in theConnectSMS
message.
- The
Both the sender
and destination
block may use the following
sentinel replacements:
Sentinel | Replacement Type | Description |
---|---|---|
vlr |
Full | The full VLR number from the InitialDPSMS message. |
vlr_cc |
Prefix | The E.164 country code of the VLR number from the InitialDPSMS . |
msc |
Full | The full MSC address from the InitialDPSMS message. |
msc_cc |
Prefix | The E.164 country code of the MSC address from the InitialDPSMS . |
smsc |
Full | The full SMSC address from the InitialDPSMS message. |
smsc_cc |
Prefix | The E.164 country code of the SMSC address from the InitialDPSMS . |
Note that the VLR number, MSC address, and SMSC address fields are not normalised and are expected to be in E.164 international format when received.
Script Entry Parameters (InitialDPSMS Request)
The Lua script defines the service processing steps, such as the following:
local n2svcd = require "n2.n2svcd"
local idpsms_service = require "n2.n2svcd.idpsms_service"
local args = ...
n2svcd.debug ("RECEIVED InitialDPSMS")
n2svcd.debug_var (args)
local scenario = string.sub (args.initialdpsms.callingPartyNumber_digits, -2)
-- This will return ContinueSMS
if (scenario == "01") then
idpsms_service.termination_final ()
-- This will crash out and return InitialDPSMS-Error (Error Code 11)
elseif (scenario == "02") then
error ("Deliberate Error")
-- This will return ReleaseCall with cause 7
elseif (scenario == "03") then
return 7
-- This will return ReleaseCall with cause default (= 1)
elseif (scenario == "04") then
return
-- This will return ReleaseCall with cause 127
elseif (scenario == "05") then
idpsms_service.release (127)
return
end
return
The service script will be executed with an args
entry parameter which is an object with the
following attributes:
Attribute | Type | Description |
---|---|---|
.remote_sccp
|
SCCP Address Object | The far-end SCCP address, as per the TCAP-RECV Message. |
.local_sccp
|
SCCP Address Object | The near-end SCCP address, as per the TCAP-RECV Message. |
.collected_info
|
1
|
This value is present only when the InitialDPSMS has eventTypeSMS = 1 (Collected Info).
|
.delivery_requested
|
1
|
This value is present only when the InitialDPSMS has eventTypeSMS = 11 (Delivery Requested).
|
.normalised_calling_party
|
Digit String |
(Normalised) Calling Party Number address digits. This is the calling party address digits after any configured normalisation rules have been applied. It is determined from the callingPartyNumber element of the InitialDPSMS.It is guaranteed to contain only 0-9a-c*# digits.
|
.normalised_called_party
|
Digit String |
(Normalised) Called Party Number address digits. This is the called party address digits after any configured normalisation rules have been applied. It is determined from the destinationSubscriberNumber element of the InitialDPSMS.It is guaranteed to contain only 0-9a-c*# digits.
|
.normalised_logical_party
|
Digit String |
(Normalised) Logical Party address digits. This field contains the normalised address digits for the logical subscriber. The logical subscriber is the party that would be charged for the SMS. When collected_info is 1 this will be the same as normalised_calling_party .When delivery_requested is 1 this will be the same as normalised_called_party .It is guaranteed to contain only 0-9a-c*# digits.
|
.normalised_other_party
|
Digit String |
(Normalised) Other Party address digits. This field contains the normalised address digits for the party who received the SMS from a charging perspective. When collected_info is 1 this will be the same as normalised_called_party .When delivery_requested is 1 this will be the same as normalised_calling_party .It is guaranteed to contain only 0-9a-c*# digits.
|
.pending_tn
|
Digit String | The normalised pending termination number inferred from the InitialDPSMS. |
.initialdpsms
|
Object |
The decoded attributes of the InitialDPSMS operation, populated as per the relevant ASN.1 for
the INAP variant in use, either
CAMEL3 InitialDPSMS or
CAMEL4 InitialDPSMS.
|
Script Return Parameters (InitialDPSMS Response)
The Lua script is responsible for determing the message(s) to send in reply to the received
InitialDPSMS
operation.
The service provides a short-cut mechanism for doing this. If the service has not yet
performed an idpsms_service
API action such as termination_final ()
or release ()
then
returning from the script will cause a ReleaseSMS
operation to be sent back in TCAP END,
exactly as if the release ()
method has been called.
If the return value is an integer in the range 0
-127
then this will be used as the release
cause. If not specified, then the service default value will be used.
Example (returning a ReleaseSMS with cause 7):
local n2svcd = require "n2.n2svcd"
local args = ...
return 7
The InitialDPSMSLuaService API
The InitialDPSMS Service API can be loaded as follows.
local idpsms_service = require "n2.n2svcd.idpsms_service"
This will provide access the following methods and constants.
.release [Synchronous]
This method will cause the ReleaseSMS
CAMEL operation to be returned as Invoke
in TCAP END.
This instructs the SMSC to deny the SMS submission or delivery.
The InitialDPSMS transaction is over.
Subsequent calls to other InitialDPSMS Service API methods are not permitted, and will generate a runtime script error.
The release
method takes the following parameters.
Parameter | Type | Description |
---|---|---|
cause
|
0 - 127
|
The numeric cause value to include.(Default = 1 )
|
The release
method returns true
.
[Fragment] Example ReleaseSMS specifying cause:
...
idpsms_service.release (3)
[post-processing logic after InitialDPSMS transaction is ended]
...
.termination_final [Synchronous]
This method will cause the ConnectSMS
/ContinueSMS
CAMEL operation to be returned as Invoke
in TCAP END.
This instructs the SMSC to continue with the SMS submission or delivery.
The InitialDPSMS transaction is over. The service logic does not arm any event detection points, and will not be subsequently informed if the delivery is successful or not.
Subsequent calls to other InitialDPSMS Service API methods are not permitted, and will generate a runtime script error.
The termination_final
method takes the following parameters.
Parameter | Type | Description |
---|---|---|
destination
|
String |
The destination subscriber digits. If provided, will force a ConnectSMS message to be used.
Otherwise, a ContinueSMS message will be used.(Default = not populated, a ContinueSMS message will be used)
|
calling
|
String |
The calling subscriber digits to be sent in the ConnectSMS message.
May not be specified without destination .(Default = not populated) |
smsc
|
String |
The SMSC address to be sent in the ConnectSMS message.
May not be specified without destination .(Default = not populated) |
The termination_final
method returns true
.
[Fragment] Example SMS termination without arming EDPs:
...
idpsms_service.termination_final ()
[post-processing logic after InitialDPSMS transaction is ended]
...
.termination_attempt [Asynchronous]
This method will cause the RequestReportSMSEvent
and ConnectSMS
/ContinueSMS
CAMEL operations to be returned as Invoke
components in TCAP CONTINUE.
This instructs the SMSC to continue with the SMS submission (SMS-MO) or delivery (SMS-MT) and report the success/failure of this attempt.
For SMS submission, the EDPs 2 (failure) and 3 (submitted) will be armed. For SMS delivery, the EDPs 12 (failure) and 13 (delivered) will be armed.
The service logic will suspend until a response is received - being either:
- A
EventReportSMS
operation, or (in error cases) - a
ReturnError
component, or - a returned TCAP ABORT, or
- a returned empty TCAP END message.
The service will also start a timer. If none of the above responses are returned then the termination attempt
will be terminated according to the configured timeout_policy
, and return with Reason = Timeout
. Note
that this library does not support use of the ResetTimer
operation, and so the timer duration
must be a value agreed with the SMSC as being acceptable within the maximum TCAP transaction timers.
An all return cases, subsequent calls to other InitialDPSMS Service API methods are not permitted, and will generate a runtime script error.
The termination_attempt
method takes the following parameters.
Parameter | Type | Description |
---|---|---|
seconds
|
Number |
The duration of the timer in seconds. (Default = the service configured default value) |
destination
|
String |
The destination subscriber digits. If provided, will force a ConnectSMS message to be used.
Otherwise, a ContinueSMS message will be used.(Default = not populated, a ContinueSMS message will be used)
|
calling
|
String |
The calling subscriber digits to be sent in the ConnectSMS message.
May not be specified without destination .(Default = not populated) |
smsc
|
String |
The SMSC address to be sent in the ConnectSMS message.
May not be specified without destination .(Default = not populated) |
The termination_attempt
method returns the following object structure:
Parameter | Type | Description |
---|---|---|
result
|
Abandon / Failure / Submitted / Delivered / Error / Timeout / Abort
|
Indicates the reason for the completion of the operation. |
ok
|
Boolean |
A convenience flag which is true iff result is = Submitted or Delivered .
|
[Fragment] Example SMS termination with armed EDPs:
...
local result = idpsms_service.termination_attempt (20.0)
if (result.ok) then
diameter_lib.commit_charge ()
else
diameter_lib.revert_charge ()
end
...
Constants
The following constants define the supported values for the result.reason
field returned from the .termination_attempt
method.
-- Our possible result.reason values (for methods above).
idpsms_service.REASON_FINAL = 'Final' -- We sent ReleaseSMS OR ConnectSMS/ContinueSMS (without RequestReportSMSEvent).
idpsms_service.REASON_ABANDON = 'Abandon' -- The SMSC abandoned the transaction by sending empty TCAP END instead of EventReportSMS.
idpsms_service.REASON_FAILURE = 'Failure' -- The SMSC reported failure to collect or failure to deliver.
idpsms_service.REASON_SUBMITTED = 'Submitted' -- The SMSC reported that the message was submitted.
idpsms_service.REASON_DELIVERED = 'Delivered' -- The SMSC reported that the message was delivered.
idpsms_service.REASON_ERROR = 'Error' -- The SMSC returned an ReturnError component.
idpsms_service.REASON_TIMEOUT = 'Timeout' -- The SMSC did not report any result within the allowed timeout period.
idpsms_service.REASON_ABORT = 'Abort' -- The SMSC abandoned the transaction by sending TCAP ABORT instead of EventReportSMS.