Diameter Lua Agent
Introduction
The DiameterLuaAgent is an asynchronous helper for Lua scripts running within the LogicApp. It is used for sending out Diameter client requests.
- The Diameter Agent may be used by any Lua script, regardless of which Service initiated that script.
The DiameterLuaAgent communicates with one or more instances of the DiameterApp which can be used to communicate with one or more external Diameter servers.
The DiameterLuaAgent communicates with the DiameterApp using the DIAMETER-C-… messages.
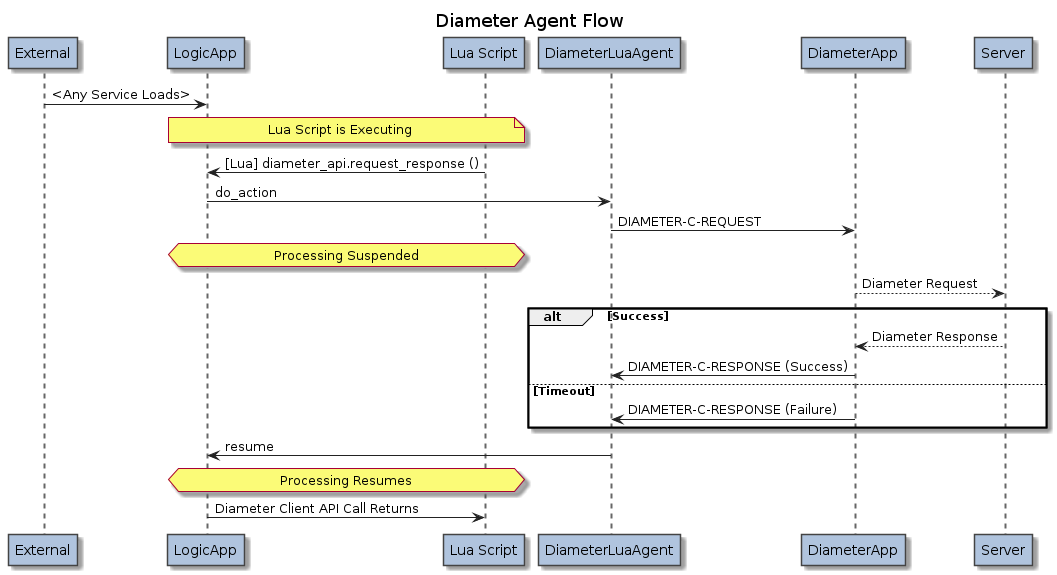
Diameter Agent API methods are accessed via the “n2.n2svcd.diameter_agent” module:
local diameter_api = require "n2.n2svcd.diameter_agent"
Configuring DiameterLuaAgent
The DiameterLuaAgent is configured within a LogicApp.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
...
<parameter name="default_diameter_app_name" value="DIAMETER-CLIENT"/>
<parameter name="diameter_app_name_sms" value="DIAMETER-CLIENT-SMS"/>
</parameters>
<config>
<services>
...
</services>
<agents>
<agent module="DiameterApp::DiameterLuaAgent" libs="../apps/diameter/lib"/>
</agents>
</config>
</application>
...
</application>
...
</n2svcd>
Under normal installation, following agent
attributes apply:
Parameter Name | Type | XML Type | Description |
---|---|---|---|
module
|
DiameterApp::
DiameterLuaAgent
|
Attribute | [Required] The module name containing the Lua Agent code. |
libs
|
../apps/diameter/lib
|
Attribute |
Location of the module for DiameterLuaAgent.
|
In addition, the DiameterLuaAgent must be configured with the name of the DiameterApp with which
it will communicate. This is configured within the parameters
of the containing
LogicApp
.
Parameter Name | Type | XML Type | Description |
---|---|---|---|
parameters
|
Array | Element |
Array of name = value Parameters for this Application instance.
|
.default_diameter_app_name
|
String | Attribute | Default name for the DiameterApp with which DiameterLuaAgent will communicate. |
.diameter_app_name_<route>
|
String | Attribute |
Use this format when DiameterLuaAgent will communicate with more than one DiameterApp .
|
The DiameterLuaAgent API
All methods may raise a Lua Error in the case of exception, including:
- Invalid input parameters supplied by Lua script.
- Unexpected results structure returned from DiameterApp.
- Processing error occurred at DiameterApp.
- Timeout occurred at DiameterApp.
.request_response [Asynchronous]
The request_response
method sends a Diameter request, and returns the response. The following parameters are accepted.
Parameter | Type | Description |
---|---|---|
route
|
String |
Optional Diameter route to use to submit the message. If undefined the default route will be used. (Default = undef )
|
command
|
String or Integer | The String command name or Integer command code of the command request to send. |
avps
|
Array of Object | Array of AVP descriptors each with the following fields. |
[].bytes
|
String |
Optional raw bytes representing the entire AVP including headers and padding. If you supply this, then it is used without further checking, and all other attributes are ignored. |
[].name
|
String |
The name of the Diameter AVP, e.g. Rating-Group .This must be the name of a supported AVP within N2::DIAMETER::Codec or DiameterApp custom AVPs.If your AVP is not supported, you may instead specify [].code .
|
[].code
|
Integer |
The code of the Diameter AVP, if not specifying by [].name .
|
[].vendor_id
|
Integer |
The vendor ID. This field is optional and can be specified both when
selecting the AVP by [].name (to distinguish between AVPs which have the same name
but which are specified by different vendors) or when selecting the AVP by [].code
in order to specify the intended vendor ID.
|
[].type
|
String |
One of None , OctetString , UTF8String , DiamIdent , Integer32 ,
Integer64 , Unsigned32 , Enumerated , Time , Unsigned64 ,
Address , Grouped .This is used only when specifying an AVP by [].code .For named AVPs the type is pre-defined. |
[].mandatory
|
0 /1
|
Override the default mandatory AVP flag. This is used only when specifying an AVP by [].code .For named AVPs the mandatory flag is pre-defined. |
[].encryption
|
0 /1
|
Override the default encryption AVP flag. This is used only when specifying an AVP by [].code .For named AVPs the encryption flag is pre-defined. |
[].value
|
Various |
The value for this AVP. For AVPs of type Grouped this will be an ARRAY of AVPs.For all other AVPs this is a SCALAR of the appropriate type. |
auto_session_id
|
0 /1
|
Flag to indicate if the Session-Id AVP should be automatically assigned. (Default = 0 )
|
The request_response
method returns a Diameter response
object with the following fields.
Field | Type | Description |
---|---|---|
command
|
String | The String command name if known. |
code
|
Integer | The Integer command code of the received response. |
session_id
|
String | The String session ID associated with the current Diameter context if it was assigned by `auto_session_id` in the inbound request. |
avps
|
Array of Object | Array of AVP descriptors each with the fields described as for a request. |
Example (Diameter Client Request):
local n2svcd = require "n2.n2svcd"
local diameter_api = require "n2.n2svcd.diameter_agent"
local soap_args = ...
local result = diameter_api.request_response ('sms', 'Credit-Control-Request', {
{ name = "Service-Context-Id", value = "sms@huawei.com" },
{ name = "CC-Request-Type", value = 1 },
{ name = "CC-Request-Number", value = 1 },
{ name = "Rating-Group", value = 900 },
{ name = "Requested-Action", value = 0 },
{ name = "Requested-Service-Unit",
value = { { name = "CC-Service-Specific-Units", value = 1 } },
vendor_specific = 0
},
{ name = "Service-Identifier", value = 200 },
{ name = "Subscription-Id",
value = {
{ name = "Subscription-Id-Data", value = "64220635462" },
{ name = "Subscription-Id-Type", value = 0 }
}
},
{ name = "Custom-1", value = 789 }
}, true)
soap_response = {}
soap_response['status'] = 'ok'
return soap_response
The above example shows a LogicApp
script invoked by a SoapLuaService.
Note that the decoded .avps
array will contain all fields, i.e. the [].name
and [].code
and [].type
and [].mandatory
, etc. will all be present.
The only exception will be when decoding AVPs which are not part of the core fields
defined within N2::DIAMETER::Codec
and are not defined as custom AVPs in the
configuration for DiameterApp
. In these cases, the [].name
and [].type
AVP attributes will not be present, and the [].value
will be the raw undecoded
bytes of the AVP value without any interpretation.
.get_first_instance_of_avp [Synchronous]
The get_first_instance_of_avp
method searches an array of AVPs, returning the first AVP with name matching a specified name. The following parameters are accepted.
Parameter | Type | Description |
---|---|---|
avps
|
Array of Object | Array of AVP descriptors each with the fields described for the result. |
name
|
String | The name of the AVP to search for. |
If a matching AVP is found, the get_first_instance_of_avp
method returns an avp
object with the following fields.
Field | Type | Description |
---|---|---|
.name
|
String |
The name of the Diameter AVP, e.g. Rating-Group .This field will only be present if the AVP is included within N2::DIAMETER::Codec or DiameterApp custom AVPs.
|
.code
|
Integer | The code that identifies the Diameter AVP. |
.vendor_id
|
Integer | The identifier of the vendor that defines the AVP if the AVP is a vendor-specific AVP. |
.type
|
String |
One of None , OctetString , UTF8String , DiamIdent , Integer32 ,
Integer64 , Unsigned32 , Enumerated , Time , Unsigned64 ,
Address , Grouped .This field will only be present if the AVP is included within N2::DIAMETER::Codec or DiameterApp custom AVPs.
|
.mandatory
|
0 /1
|
The value of the AVP's mandatory flag. |
.encryption
|
0 /1
|
The value of the AVP's encryption flag. |
.value
|
Various |
The AVP's value. For AVPs of type Grouped this will be an ARRAY of AVPs.For all other AVPs this is a SCALAR of the appropriate type. |
.get_first_value_of_avp [Synchronous]
The get_first_value_of_avp
method searches an array of AVPs, returning the value of the first AVP with name matching a specified name. The following parameters are accepted.
Parameter | Type | Description |
---|---|---|
avps
|
Array of Object |
Array of AVP descriptors each with the fields described for the get_first_instance_of_avp result.
|
name
|
String | The name of the AVP to search for. |
If a matching AVP is found, the get_first_value_of_avp
method returns that AVP’s value.
For AVPs of type Grouped
this will be an ARRAY of AVPs.
For all other AVPs this is a SCALAR of the appropriate type.
.get_all_instances_of_avp [Synchronous]
The get_all_instances_of_avp
method searches an array of AVPs, returning all AVPs with name matching a specified name. The following parameters are accepted.
Parameter | Type | Description |
---|---|---|
avps
|
Array of Object |
Array of AVP descriptors each with the fields described for the get_first_instance_of_avp result.
|
name
|
String | The name of the AVP(s) to search for. |
If at least one matching AVP is found, the get_all_instances_of_avp
method returns an array of AVPs as per get_first_instance_of_avp
.
Otherwise an empty array is returned.
Built-In N2::Diameter::Codec Commands
The following list of Command names is built-in to the N2::Diameter::Codec
encoding
and decoding library. Commands not in this list may be referenced by numeric code,
although they will not have support for setting special flags, or for changing the
Diameter Application ID.
Name | Code |
---|---|
AA | 265 |
Abort-Session | 274 |
Accounting | 271 |
Authentication-Information | 318 |
Bootstrapping-Info | 310 |
Capabilities-Exchange | 257 |
Credit-Control | 272 |
Device-Watchdog | 280 |
Diameter-EAP | 268 |
Disconnect-Peer | 282 |
Location-Info | 302 |
Message-Process | 311 |
Multimedia-Auth | 303 |
Notify | 323 |
Profile-Update | 307 |
Push-Notification | 309 |
Push-Profile | 305 |
Re-Auth | 258 |
Registration-Termination | 304 |
Server-Assignment | 301 |
Session-Termination | 275 |
Spending-Limit | 8388635 |
Spending-Status-Notification | 8388636 |
Subscribe-Notifications | 308 |
Update-Location | 316 |
User-Authorization | 300 |
User-Data | 306 |
Built-In N2::Diameter::Codec AVPs
The following list of AVPs is built-in to the N2::Diameter::Codec
encoding and
decoding library. Additional custom AVPs may be added as necessary on a
per-site basis by using configuration in the
DiameterApp.
Name | Code | Type & Flags |
---|---|---|
User-Name | 1 | UTF8String, Mandatory |
Class | 25 | OctetString, Mandatory |
Session-Timeout | 27 | Unsigned32, Mandatory |
Proxy-State | 33 | OctetString, Mandatory |
Accounting-Session-Id | 44 | OctetString, Mandatory |
Acct-Multi-Session-Id | 50 | UTF8String, Mandatory |
Event-Timestamp | 55 | Time, Mandatory |
Acct-Interim-Interval | 85 | Unsigned32, Mandatory |
Host-IP-Address | 257 | Address, Mandatory |
Auth-Application-Id | 258 | Unsigned32, Mandatory |
Acct-Application-Id | 259 | Unsigned32, Mandatory |
Vendor-Specific-Application-Id | 260 | Grouped, Mandatory |
Redirect-Host-Usage | 261 | Enumerated, Mandatory |
Redirect-Max-Cache-Time | 262 | Unsigned32, Mandatory |
Session-Id | 263 | UTF8String, Mandatory |
Origin-Host | 264 | DiamIdent, Mandatory |
Supported-Vendor-Id | 265 | Unsigned32, Mandatory |
Vendor-Id | 266 | Unsigned32, Mandatory |
Firmware-Revision | 267 | Unsigned32 |
Result-Code | 268 | Unsigned32, Mandatory |
Product-Name | 269 | UTF8String |
Session-Binding | 270 | Unsigned32, Mandatory |
Session-Server-Failover | 271 | Enumerated, Mandatory |
Multi-Round-Time-Out | 272 | Unsigned32, Mandatory |
Disconnect-Cause | 273 | Enumerated, Mandatory |
Auth-Request-Type | 274 | Enumerated, Mandatory |
Auth-Grace-Period | 276 | Unsigned32, Mandatory |
Auth-Session-State | 277 | Enumerated, Mandatory |
Origin-State-Id | 278 | Unsigned32, Mandatory |
Failed-AVP | 279 | Grouped, Mandatory |
Proxy-Host | 280 | DiamIdent, Mandatory |
Error-Message | 281 | UTF8String |
Route-Record | 282 | DiamIdent, Mandatory |
Destination-Realm | 283 | DiamIdent, Mandatory |
Proxy-Info | 284 | Grouped, Mandatory |
Re-Auth-Request-Type | 285 | Enumerated, Mandatory |
Accounting-Sub-Session-Id | 287 | Unsigned64, Mandatory |
Authorization-Lifetime | 291 | Unsigned32, Mandatory |
Redirect-Host | 292 | DiamURI, Mandatory |
Destination-Host | 293 | DiamIdent, Mandatory |
Error-Reporting-Host | 294 | DiamIdent |
Termination-Cause | 295 | Enumerated, Mandatory |
Origin-Realm | 296 | DiamIdent, Mandatory |
Experimental-Result | 297 | Grouped, Mandatory |
Experimental-Result-Code | 298 | Unsigned32, Mandatory |
Inband-Security-Id | 299 | Unsigned32, Mandatory |
E2E-Sequence | 300 | Grouped, Mandatory |
Accounting-Record-Type | 480 | Enumerated, Mandatory |
Accounting-Realtime-Required | 483 | Enumerated, Mandatory |
Accounting-Record-Number | 485 | Unsigned32, Mandatory |
Called-Station-Id | 30 | UTF8String, Mandatory |
Calling-Station-Id | 31 | UTF8String, Mandatory |
CC-Correlation-Id | 411 | OctetString |
CC-Input-Octets | 412 | Unsigned64, Mandatory |
CC-Money | 413 | Grouped, Mandatory |
CC-Output-Octets | 414 | Unsigned64, Mandatory |
CC-Request-Number | 415 | Unsigned32, Mandatory |
CC-Request-Type | 416 | Enumerated, Mandatory |
CC-Service-Specific-Units | 417 | Unsigned64, Mandatory |
CC-Session-Failover | 418 | Enumerated, Mandatory |
CC-Sub-Session-Id | 419 | Unsigned64, Mandatory |
CC-Time | 420 | Unsigned32, Mandatory |
CC-Total-Octets | 421 | Unsigned64, Mandatory |
CC-Unit-Type | 454 | Enumerated, Mandatory |
Check-Balance-Result | 422 | Enumerated, Mandatory |
Cost-Information | 423 | Grouped, Mandatory |
Cost-Unit | 424 | UTF8String, Mandatory |
Credit-Control | 426 | Enumerated, Mandatory |
Credit-Control-Failure-Handling | 427 | Enumerated, Mandatory |
Currency-Code | 425 | Unsigned32, Mandatory |
Direct-Debiting-Failure-Handling | 428 | Enumerated, Mandatory |
Exponent | 429 | Integer32, Mandatory |
Final-Unit-Action | 449 | Enumerated, Mandatory |
Final-Unit-Indication | 430 | Grouped, Mandatory |
Granted-Service-Unit | 431 | Grouped, Mandatory |
G-S-U-Pool-Identifier | 453 | Unsigned32, Mandatory |
G-S-U-Pool-Reference | 457 | Grouped, Mandatory |
Multiple-Services-Credit-Control | 456 | Grouped, Mandatory |
Multiple-Services-Indicator | 455 | Enumerated, Mandatory |
Rating-Group | 432 | Unsigned32, Mandatory |
Redirect-Address-Type | 433 | Enumerated, Mandatory |
Redirect-Server | 434 | Grouped, Mandatory |
Redirect-Server-Address | 435 | UTF8String, Mandatory |
Requested-Action | 436 | Enumerated, Mandatory |
Requested-Service-Unit | 437 | Grouped, Mandatory |
Restriction-Filter-Rule | 438 | IPFiltrRule, Mandatory |
Service-Context-Id | 461 | UTF8String, Mandatory |
Service-Identifier | 439 | Unsigned32, Mandatory |
Service-Parameter-Info | 440 | Grouped |
Service-Parameter-Type | 441 | Unsigned32 |
Service-Parameter-Value | 442 | OctetString |
Subscription-Id | 443 | Grouped, Mandatory |
Subscription-Id-Data | 444 | UTF8String, Mandatory |
Subscription-Id-Type | 450 | Enumerated, Mandatory |
Tariff-Change-Usage | 452 | Enumerated, Mandatory |
Tariff-Time-Change | 451 | Time, Mandatory |
Unit-Value | 445 | Grouped, Mandatory |
Used-Service-Unit | 446 | Grouped, Mandatory |
User-Equipment-Info | 458 | Grouped |
User-Equipment-Info-Type | 459 | Enumerated |
User-Equipment-Info-Value | 460 | OctetString |
Value-Digits | 447 | Integer64, Mandatory |
Validity-Time | 448 | Unsigned32, Mandatory |
Subscription-Id-Extension | 659 | Grouped, Mandatory |
Subscription-Id-E164 | 660 | UTF8String, Mandatory |
Subscription-Id-IMSI | 661 | UTF8String, Mandatory |
Subscription-Id-SIP-URI | 662 | UTF8String, Mandatory |
Subscription-Id-NAI | 663 | UTF8String, Mandatory |
Subscription-Id-Private | 664 | UTF8String, Mandatory |
Vendor ID = 10415 | ||
3GPP-IMSI | 1 | UTF8String |
3GPP-GGSN-Address | 7 | OctetString |
3GPP-GGSN-MCC-MNC | 9 | UTF8String |
3GPP-SGSN-MCC-MNC | 18 | UTF8String |
3GPP-RAT-Type | 21 | OctetString |
3GPP-User-Location-Info | 22 | OctetString |
3GPP-MS-TimeZone | 23 | OctetString |
Policy-Counter-Identifier | 2901 | UTF8String, Mandatory |
Policy-Counter-Status | 2902 | UTF8String, Mandatory |
Policy-Counter-Status-Report | 2903 | Grouped, Mandatory |
SL-Request-Type | 2904 | Enumerated, Mandatory |
Event-Type | 823 | Grouped, Mandatory |
Event | 825 | UTF8String, Mandatory |
Calling-Party-Address | 831 | UTF8String, Mandatory |
Called-Party-Address | 832 | UTF8String, Mandatory |
Application-Provided-Called-Party-Address | 837 | UTF8String, Mandatory |
GGSN-Address | 847 | Address, Mandatory |
Application-Server-Information | 850 | Grouped, Mandatory |
Time-Quota-Threshold | 868 | Unsigned32, Mandatory |
Volume-Quota-Threshold | 869 | Unsigned32, Mandatory |
Service-Information | 873 | Grouped, Mandatory |
PS-Information | 874 | Grouped, Mandatory |
IMS-Information | 876 | Grouped, Mandatory |
LCS-Information | 878 | Grouped, Mandatory |
Address-Data | 897 | UTF8String, Mandatory |
Address-Type | 899 | Enumerated, Mandatory |
QoS-Information | 1016 | Grouped |
Recipient-Address | 1201 | Grouped, Mandatory |
SGSN-Address | 1228 | Address, Mandatory |
Requested-Party-Address | 1251 | UTF8String, Mandatory |
SMS-Information | 2000 | Grouped, Mandatory |
SM-Message-Type | 2007 | Enumerated, Mandatory |
Refund-Information | 2022 | OctetString, Mandatory |
Recipient-Info | 2026 | Grouped, Mandatory |
VCS-Information | 3410 | Grouped, Mandatory |
Bearer-Capability | 3412 | OctetString, Mandatory |
ISUP-Location-Number | 3414 | OctetString, Mandatory |
MSC-Address | 3417 | OctetString, Mandatory |
VLR-Number | 3420 | OctetString, Mandatory |
Vendor ID = 3512 | ||
ORA-Account-Topup | 206 | Grouped, Mandatory |
ORA-Recharge-Reference | 207 | UTF8String, Mandatory |
ORA-Balance | 208 | Grouped, Mandatory |
ORA-Validity-Start-Time | 213 | Time, Mandatory |
ORA-Validity-End-Time | 214 | Time, Mandatory |
ORA-Validity-Start-Relative | 215 | Grouped, Mandatory |
ORA-Validity-End-Relative | 216 | Grouped, Mandatory |
ORA-First-Usage-Validity | 217 | Grouped, Mandatory |
ORA-Validity-Offset | 218 | Unsigned32, Mandatory |
ORA-Validity-Unit | 219 | Enumerated, Mandatory |
ORA-Extend-Bucket-Validity | 228 | Enumerated, Mandatory |
ORA-Customer-Cost-Information | 231 | Grouped, Mandatory |
ORA-Cost-Information | 232 | Grouped, Mandatory |
ORA-Balance-Element-Id | 233 | Integer32, Mandatory |
ORA-Subscriber-Id | 235 | OctetString, Mandatory |
ORA-Remaining-Balance | 241 | Grouped, Mandatory |
ORA-Balance-Element | 243 | Grouped, Mandatory |
ORA-Earliest-Expiry-Time | 245 | Time, Mandatory |
ORA-Balance-Query-Mode | 248 | Integer32, Mandatory |
ORA-Balance-Details | 249 | Grouped, Mandatory |
ORA-Credit-Floor | 252 | Grouped, Mandatory |