SOAP Lua Service
Introduction
The SoapLuaService is a service for initiating Lua scripts running within the LogicApp.
The SoapLuaService receives messages from one or more instances of the SoapServerApp which is configured to receive SOAP/HTTP(S) Requests from an external client.
The SoapLuaService communicates with the SoapServerApp using the SOAP-S-… messages.
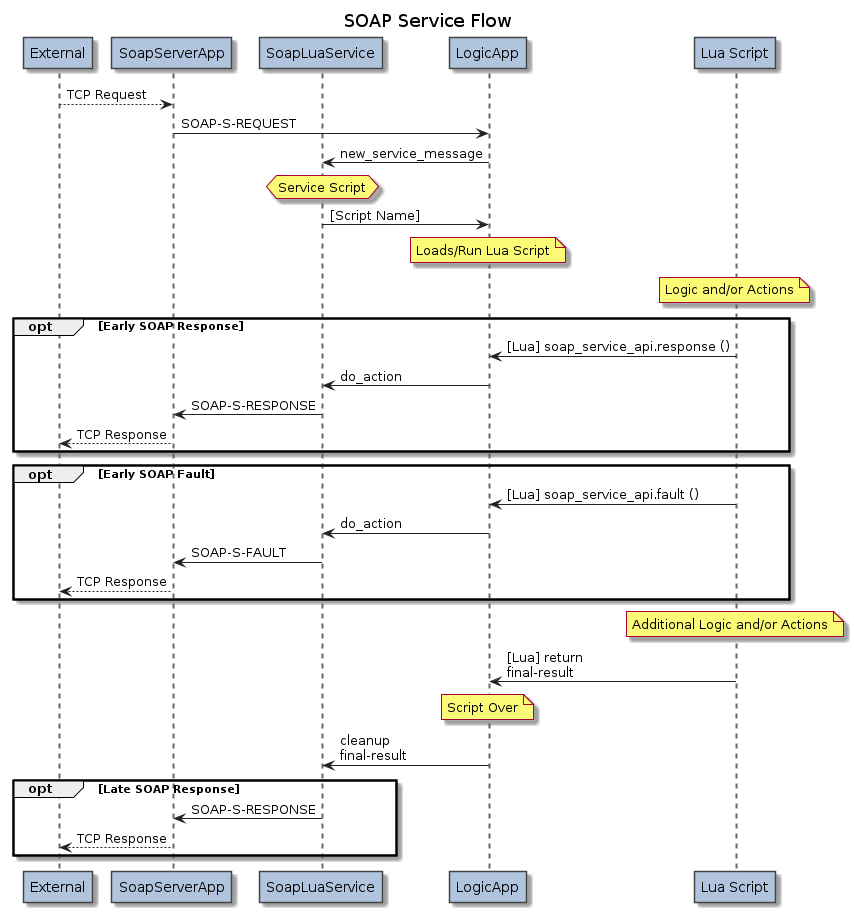
Configuring SoapLuaService
The SoapLuaService is configured within a LogicApp.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
...
</parameters>
<config>
<services>
<service module="SoapServerApp::SoapLuaService" libs="../apps/soap_s/lib" script_dir="/var/lib/n2svcd/logic/soap"/>
</services>
<agents>
...
</agents>
</config>
</application>
...
</application>
...
</n2svcd>
In addition to the Common LogicApp Service Configuration, note the following specific attribute notes, and service-specific attributes.
Under normal installation, the following service
attributes apply:
Parmeter Name | Type | XML Type | Description |
---|---|---|---|
module
|
String | Attribute |
[Required] The module name containing the Lua Service code: SoapServerApp::SoapLuaService
|
libs
|
String | Element |
Location of the module for SoapLuaService.(Default: ../apps/soap_s/lib )
|
script_dir
|
String | Attribute | [Required] The directory containing scripts used by this service. |
Script Selection (SOAP Request)
Script selection is not configured. The script name is simply the SOAP message name received in the request.
e.g. an inbound request with message name OfferList
will map to a script name of OfferList
,
which will be satisfied by a file named OfferList.lua
or OfferList.lc
in any of the directories
configured as lua_script_dir
in the LogicApp.
Refer to the LogicApp configuration for more information on directories, library paths, and script caching parameters.
Script Global Variables
Scripts run with this service have access to the Common LUA Service Global Variables.
There are no service-specific global variables.
Script Entry Parameters (SOAP Request)
The Lua script must be a Lua chunk such as the following:
local n2svcd = require "n2.n2svcd"
local soap = ...
n2svcd.debug ("Echo Supplied SOAP Inputs:")
n2svcd.debug_var (soap)
return soap.args
The chunk will be executed with a single soap
entry parameter which is an object with the
following attributes:
Field | Type | Description |
---|---|---|
.remote_ip
|
String | The remote IP address from which the SOAP HTTP(S) request was sent. |
.remote_port
|
Integer | The remote TCP/IP port from which the SOAP HTTP(S) request was sent. |
.method
|
String | The HTTP Request method. |
.uri
|
String |
The URI for which the request was received. Includes only path and query. No host/port/auth/user/fragment is present. |
.path
|
String | The URI path. |
.query
|
String | The URI query string (the part following "?" in the URI). |
.query_args
|
String or Object |
If the .query attribute contains & or = then this query_args contains a table of the
name and values, decoded according to common web form conventions. If you wish to have
full control of the decoding, then you should directly reference the undecoded .query .
|
.message_name
|
String | The name of the SOAP message (without namespace), being the name of the element contained within the SOAP body. |
.args
|
Object |
The args provided by the client into the SOAP request.
This may be a complex, nested structure.
The decoding and parsing of the args into an internal object
representation differs slightly according the quirks_mode .
|
.message_namespace
|
Object | A container for the message namespace information. |
.location
|
message /envelope
|
Location of the message namespace. |
.alias
|
String | The alias for the message namespace, if one was used. |
.url
|
String | The message namespace URL, if one was provided. |
.standard_namespaces
|
Object | A container for the standard namespace information. |
.encoding_alias
|
String | The alias for the standard encoding namespace, if present. |
.envelope_alias
|
String | The alias for the standard envelope namespace, if present. |
.schema_alias
|
String | The alias for the standard schema namespace, if present. |
.schema_instance_alias
|
String | The alias for the standard schema instance namespace, if present. |
.http_headers
|
Array of Object |
The list of HTTP headers parsed from the HTTP Request (see below for object structure). All HTTP headers are present, including Content-Length and Content-Type .If a HTTP header was repeated in the HTTP Request, then it is repeated in this Array. |
Each object in the .http_headers
Array for the soap
entry parameter has the following structure.
Field | Type | Description |
---|---|---|
.name
|
String
|
[Required] The name of the HTTP Request header. |
.value
|
String
|
[Required] The full string value of the HTTP Request header. |
Script Return Parameters (SOAP Response)
The Lua script is responsible for determing the SOAP Response which will be sent back in reply to the original SOAP Request.
The simplest way to do this is by the return value given back to the service at the end of script execution.
The script return value is simply an args
Table which will be encoded as the SOAP Body.
Field | Type | Description |
---|---|---|
args
|
Object |
The arguments which we will encode into XML for the SOAP response. The quirks_mode for the SoapServerApp will control how this is done.
|
This simple method does not allow control over the response message name, and does
not allow setting of custom HTTP header fields. You must use the .response
SOAP API
method for controlling those values.
Example (returning SOAP Response arguments):
local n2svcd = require "n2.n2svcd"
local soap = ...
return ({
ARG1 = "VALUE ONE",
ARG2ARRAY = {
'First Value',
'Second Value'
},
ARG3ARRAY = {
{
SUBITEM = 'First Item'
},
{
SUBITEM = 'Second Item'
}
}
})
The encoding of array elements will vary according to the quirks_mode
configured for
the SoapServerApp
. For example, when SoapServerApp
is configured to run in
generic
quirks mode, an array is encoded as:
<ARG2ARRAY soapenc:arrayType="xsd:string[2]" xsi:type="soapenc:Array">
<item xsi:type="xsd:string">First Value</item>
<item xsi:type="xsd:string">Second Value</item>
</ARG2ARRAY>
By comparison, in ncc_pi
quirks mode, an array is simply a repeated element, e.g.:
<ARG2ARRAY>First Value</pi:ARG2ARRAY>
<ARG2ARRAY>Second Value</pi:ARG2ARRAY>
The encoding of an array with sub-elements also differs by quirks_mode
.
The generic
quirks mode encodes the sub-elements as items:
<ARG3ARRAY xsi:type="soapenc:Array">
<item>
<SUBITEM>First Item</SUBITEM>
</item>
<item>
<SUBITEM>Second Item</SUBITEM>
</item>
</ARG3ARRAY>
By comparison, in ncc_pi
quirks mode, the array expands due to the key=value pair, but the item is omitted:
<ARG3ARRAY>
<SUBITEM>First Item</SUBITEM>
</ARG3ARRAY>
<ARG3ARRAY>
<SUBITEM>Second Item</SUBITEM>
</ARG3ARRAY>
Alternatively, a script may return nil
to return an empty SOAP response.
Example (returning a SOAP Response with no arguments):
local n2svcd = require "n2.n2svcd"
local soap = ...
return nil
The SoapLuaService API
The SOAP Service API can be loaded as follows.
local soap_service_api = require "n2.n2svcd.soap_service"
It is not necessary to load the SOAP Service API if you are only using the simple response mechanism described above. It is only required if you wish to use any of the extended features described below.
.response
When a Lua Script needs to perform extended processing, it may wish to send an early SOAP
response before the script completes. This can be done with the response
method on the
SOAP Service API. This method also allows the script to use a non-default response message name.
The response
method takes the following parameters.
Parameter | Type | Description |
---|---|---|
args
|
Integer | The SOAP arguments to be encoded into the response. |
message_name
|
String |
Response message name to use. (Default = Request name with Response appended)
|
http_headers
|
Array of Objects
|
Additional user-level header objects to apply to an outbound HTTP Response (see below for object structure). These headers are added after any headers defined by static SoapServerApp application configuration.You should not set the Content-Type or Content-Length headers.(Default = No Additional Headers) |
The response
method returns true
.
[Fragment] Example (Early Response with overridden name and extra headers):
...
soap_service_api.response (
{ num_updated = 3, new_ids = [ "1003", "3344"] },
"OfferSaveACK",
{ { name = 'Set-Cookie', value = 'FOO=BAR' },
{ name = 'Custom-HDR', value = 'success; Expiry=7' }
}
)
...
[post-processing after SOAP transaction is concluded]
...
.fault
When a Lua Script encounters a scenario where extended processing is not required and the response should generate a SOAP fault, it may wish to send an early SOAP fault before the script completes.
This can be done with the fault
method on the SOAP Service API. This method also allows the script to use a non-default fault message name.
The fault
method takes the following parameters.
Parameter | Type | Description |
---|---|---|
args
|
Integer | The SOAP arguments to be encoded into the fault. |
http_headers
|
Array of Objects
|
Additional user-level header objects to apply to an outbound HTTP Response (see below for object structure). These headers are added after any headers defined by static SoapServerApp application configuration.You should not set the Content-Type or Content-Length headers.(Default = No Additional Headers) |
The fault
method returns true
.
[Fragment] Example (Early Fault with extra headers):
...
soap_service_api.fault ({
fault_code = 400
, fault_string = 'Missing required parameter.'
, fault_actor = 'Client'
}
, {
{
name = 'Set-Cookie'
, value = 'FOO=BAR'
}
}
)
...
[post-processing after SOAP transaction is concluded]
...