RADIUS Lua Service
Introduction
The RadiusLuaService is a service for initiating Lua scripts running within the LogicApp.
The RadiusLuaService receives messages from one or more instances of the RadiusApp which is configured to receive RADIUS Requests from an external client.
The RadiusLuaService communicates with the RadiusApp using the RADIUS-S-… messages.
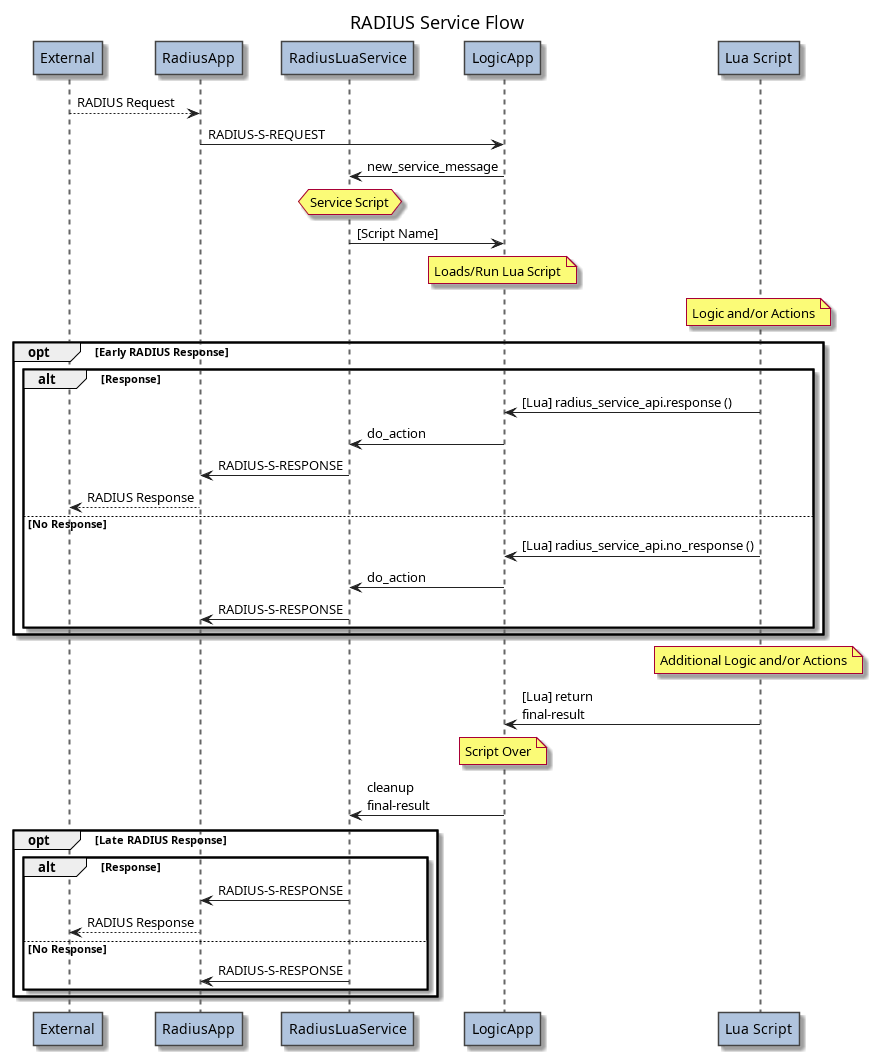
Configuring RadiusLuaService
The RadiusLuaService is configured within a LogicApp.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
...
</parameters>
<config>
<services>
<service module="RadiusApp::RadiusLuaService" libs="../apps/radius/lib" script_dir="/var/lib/n2svcd/logic/radius"/>
</services>
<agents>
...
</agents>
</config>
</application>
...
</application>
...
</n2svcd>
In addition to the Common LogicApp Service Configuration, note the following specific attribute notes, and service-specific attributes.
Under normal installation, the following service
attributes apply:
Parmeter Name | Type | XML Type | Description |
---|---|---|---|
module
|
String | Attribute |
[Required] The module name containing the Lua Service code: RadiusApp::RadiusLuaService
|
libs
|
String | Attribute |
Location of the module for RadiusLuaService.(Default: ../apps/radius/lib )
|
script_dir
|
String | Attribute | [Required] The directory containing scripts used by this service. |
Script Selection (RADIUS Request)
Script selection is not configured. The script name is simply the name or code associated with the packet type of the received RADIUS request.
e.g. an inbound Access-Request
packet will be satisfied by a file named Access-Request.lua
in
the script_dir
directory configured for the service.
Refer to the LogicApp configuration for more information on directories, library paths, and script caching parameters.
Script Global Variables
Scripts run with this service have access to the Common LUA Service Global Variables.
There are no service-specific global variables.
Script Entry Parameters (RADIUS Request)
The Lua script must be a Lua chunk such as the following:
local n2svcd = require "n2.n2svcd"
local radius = ...
n2svcd.debug ("Echo Supplied RADIUS Inputs:")
n2svcd.debug_var (radius)
-- Echo back all of the received attributes with a custom attribute appended.
radius.attributes.insert ({ type = 42, data_type = 'integer', value = 42 })
return radius
The chunk will be executed with a single radius
entry parameter which is an object representing
the received request, with the following attributes:
Field | Type | Description |
---|---|---|
.name
|
String |
The name of the RADIUS packet type, e.g. Access-Request .This field will only be populated if the packet type is supported within N2::RADIUS::Codec .
|
.code
|
Integer | [Required] The packet type identifier from the received request. |
.identifier
|
Integer | [Required] The identifier field from the received request. |
.length
|
Integer | [Required] The length field from the received request. |
.authenticator
|
String | [Required] The authenticator field from the received request. |
.attributes
|
Array of Object | Array of RADIUS attribute objects in the request, each with the following fields. |
[].name
|
String |
The name of the RADIUS attribute, e.g. User-Name .This field will only be populated if the attribute is supported within N2::RADIUS::Codec .
|
[].type
|
Integer | [Required] The code that identifies the RADIUS attribute. |
[].vendor_id
|
Integer |
The identifier of the vendor that defines the attribute if the attribute is a vendor-specific attribute. This field will only be present if the attribute is supported in N2::RADIUS::Codec .
|
[].data_type
|
String |
[Required] One of enum , ifid , integer , ipv4addr , ipv4prefix ,
ipv6addr , ipv6prefix , string , other , text ,
time , unknown .
|
[].value
|
Various |
[Required] The attribute's value. For most attributes this will be a SCALAR of the appropriate type. For attributes with data type other this may be a table.
|
[].value_name
|
String |
The name associated with [].value .This field will only be present if the attribute [].data_type is enum and the value mapping is defined in N2::RADIUS::Codec .
|
Script Return Parameters (RADIUS Response)
The Lua script is responsible for determing the RADIUS Response which will be sent back in reply to the original RADIUS Request.
The simplest way to do this is by the return value given back to the service at the end of script execution.
To indicate that no response should be sent, the script should return an empty table.
To send a response, the script should return a table containing the following attributes.
Field | Type | Description |
---|---|---|
.name
|
String |
The RADIUS packet type name of the response to send, e.g. Access-Accept .This must be the name of a supported packet type within N2::RADIUS::Codec .If the packet type is not supported, you may instead specify .code .
|
.code
|
Integer |
The RADIUS packet type code, e.g. 2 for Access-Accept .
|
.attributes
|
Array of Object | Array of RADIUS attribute objects to include in the response, each with the following fields. |
[].name
|
String |
The name of a RADIUS attribute, e.g. User-Name .This must be the name of a supported attribute within N2::RADIUS::Codec .If the attribute is not supported, you may instead specify [].type and [].data_type .
|
[].type
|
Integer | The code that identifies the RADIUS attribute. |
[].vendor_id
|
Integer |
The identifier of the vendor that defines the attribute if the attribute is a vendor-specific attribute. This field is optional and can be used to disambiguate attribute definitions when [].name and/or [].type are not unique.
|
[].data_type
|
String |
One of enum , ifid , integer , ipv4addr , ipv4prefix ,
ipv6addr , ipv6prefix , string , other , text ,
time .This field is used only when the attribute is not supported in N2::RADIUS::Codec .
For supported attributes the data type is pre-defined.
|
[].value
|
Various |
The attribute's value. For most attributes this will be a SCALAR of the appropriate type. For attributes with data type other this may be a table.
|
[].value_name
|
String |
This field can be used to specify the value by constant name for attributes with [].data type value enum that have their value mapping defined in N2::RADIUS::Codec .
|
Example (returning RADIUS Response arguments):
local n2svcd = require "n2.n2svcd"
local radius = ...
return {
name = "Access-Accept",
attributes = {
{ name = "Service-Type", value_name = "Framed" },
{ name = "Custom-1", type = 42, data_type = "Integer", value = 789 }
}
}
The RadiusLuaService API
The RADIUS Service API can be loaded as follows.
local radius_service_api = require "n2.n2svcd.radius_service"
It is not necessary to load the RADIUS Service API if you are only using the simple response mechanism described above. It is only required if you wish to use any of the early response functions described below.
A chunk should return nil
after an early response. The actual return result is
ignored in that scenario.
.response
When a Lua Script needs to perform extended processing, it may wish to send an early RADIUS
response before the script completes. This can be done with the response
method on the
RADIUS Service API.
The response
method accepts the following parameters.
Parameter | Type | Description |
---|---|---|
packet_type
|
String or Integer |
[Required] The String packet type name or Integer packet type code of the response to send. If specifying a packet type name, this must be the name of a supported packet type within N2::RADIUS::Codec .
|
attributes
|
Array of Object | Array of attribute objects, each with the fields previously described for the response mechanism. |
The response
method returns true
.
[Fragment] Example (Early Response):
...
radius_service_api.response (
"Access-Accept",
{
{ name = "Service-Type", value_name = "Framed" },
{ name = "Custom-1", type = 42, data_type = "Integer", value = 789 }
}
)
...
[post-processing after RADIUS transaction is concluded]
...
return nil
.no_response
When a Lua Script needs to perform extended processing, it may wish to discard the RADIUS
request before the script completes. This can be done with the no_response
method on the
RADIUS Service API.
The no_response
method has no parameters.
The no_response
method returns true
.
[Fragment] Example (Early No-Response):
...
radius_service_api.no_response ()
...
[post-processing after RADIUS transaction is concluded]
...
return nil